RecyclerView
類似ListView及GridView,甚至能夠取代他們
RecyclerView 需要實作 RecyclerView.Adapter及Adapter的ViewHolder
1 2 3 4 5 6 7 8 9 10 11 12
| // activity_layout.xml <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.v7.widget.RecyclerView android:id="@+id/recycleview" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
|
拉好RecyclerView元件之後,還要建立item
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| // item.xml <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center_horizontal"> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView" android:textColor="#111111" android:textSize="16sp" android:textStyle="bold" /> </LinearLayout>
|
介面都完成後,就要開始打程式囉!
- 首先建立一個RecyclerViewAdapter.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public class RecycleViewAdapter extends RecyclerView.Adapter<RecycleViewAdapter.MyViewHolder> { public RecycleViewAdapter(Context context) { this.context = context; } @Override public RecycleViewAdapter.MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { return null; } @Override public void onBindViewHolder(final RecycleViewAdapter.MyViewHolder holder, final int position) { } @Override public int getItemCount() { return 0; } class MyViewHolder extends RecyclerView.ViewHolder { public MyViewHolder(View view) { super(view); } }
|
建立好之後就一個一個來修改囉
1 2 3 4 5 6 7
| class MyViewHolder extends RecyclerView.ViewHolder { TextView tv; public MyViewHolder(View view) { super(view); tv = (TextView) view.findViewById(R.id.textView2); } }
|
完成了之後,我們需要把RecyclerViewAdapter指定給RecyclerView
1 2 3 4 5 6 7 8 9 10 11 12 13
| RecyclerView mRecyclerView = (RecyclerView) view.findViewById(R.id.recycleview); RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(this); mRecyclerView.setLayoutManager(layoutManager); RecycleViewAdapter mAdapter = new RecycleViewAdapter(this); mRecyclerView.setAdapter(mAdapter);
|
大功告成
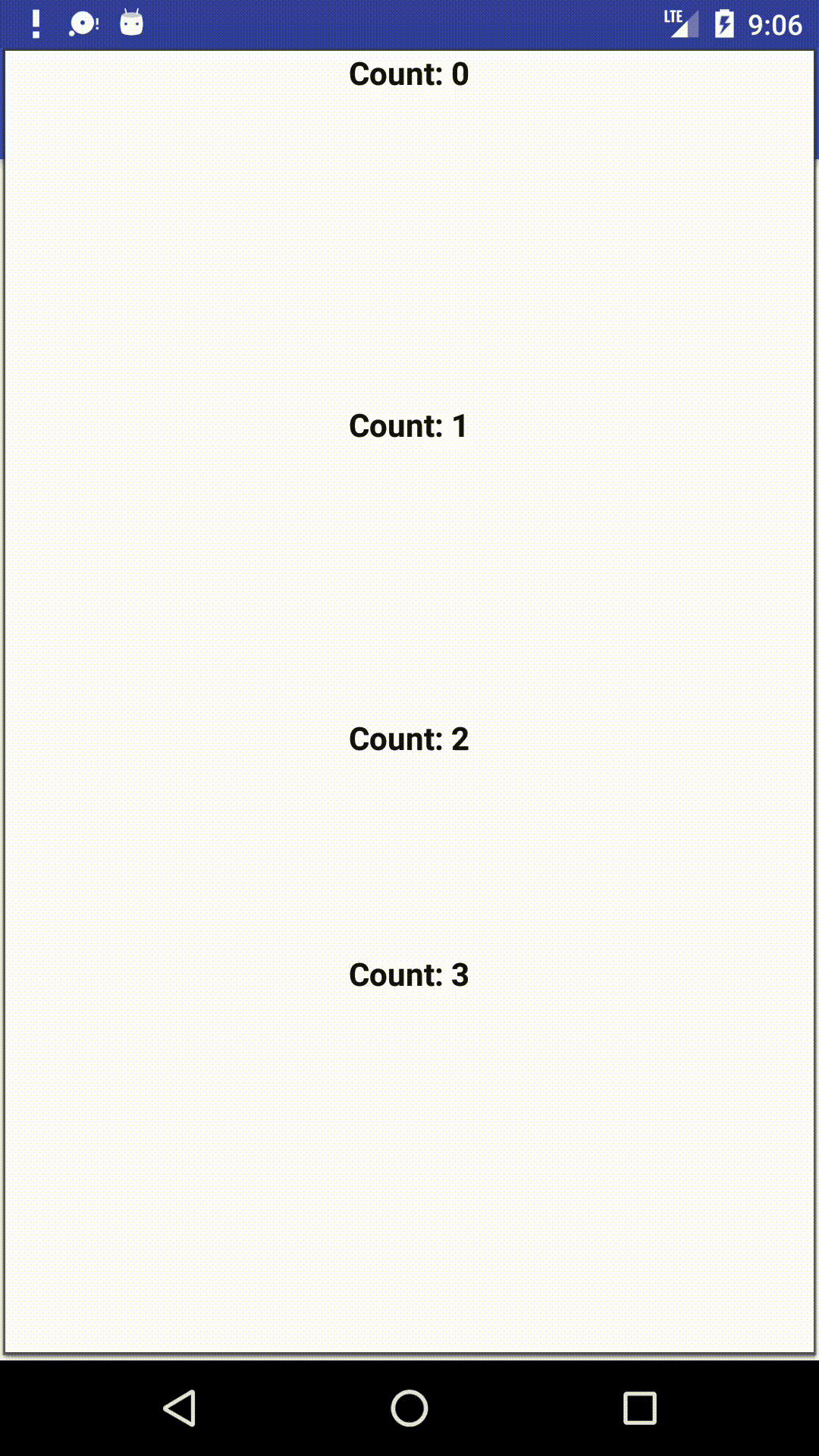